Code Snippets - Generate a random number between two numbers in JavaScript
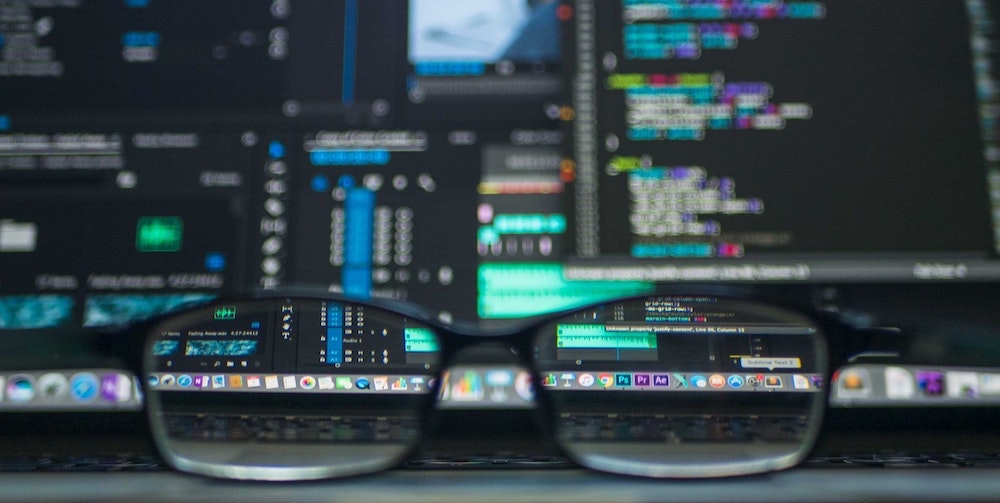
This is another blog post I write about code snippets I find useful. Below is the list of the previous code snippets I have written about:
- Sort array of objects by a property in JavaScript
- Unique array in JavaScript
- Pre-populate an array in JavaScript
- uniqueId
Today's code snippet is about how to generate a random number between two numbers in JavaScript.
I usually go for this snippet in two main scenarios:
- When prototyping a UI without an endpoint: I like to randomize stats so I don't always get to see the same results over and over.
- Unit tests so I don't create biased tests: this is how I ensure reliability for tests that need some sort of number input. It's also helpful when I need to quickly create an array and its size doesn't really matter much.
Math.random gets us halfway through there: it returns a floating-point number between 0 and 1 (exclusive).
The next step is to use Math.random
so it returns the number within the desired range:
/**
* Generates a random number between @min (inclusive) and @max (exclusive)
*/
function generateRandomNumber(min: number, max: number) {
Math.floor(Math.random() * (max - min) + min)
}
Should you need to a random number that could also be equal to max
we'd have to add a + 1
to the difference between max
and min
:
/**
* Generates a random number between @min (inclusive) and @max (inclusive)
*/
function generateRandomNumber(min: number, max: number) {
return Math.floor(Math.random() * (max - min + 1)) + min
}
I hope that's helpful and interesting to you. 👋🏼
Did you know you can help me with this page?
If you see something wrong, think this page needs clarification, you found a typo or any other suggestion you might have feel free to open a PR and I will take care of the rest.
My entire site is available to edit on GitHub and all contributions are very welcome 🤙🏼.
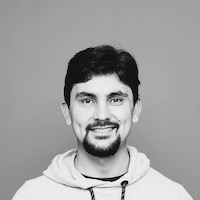
Hemerson Carlin, also known as mersocarlin, is passionate and resourceful full-stack Software Engineer with 10+ years of experience focused on agile development, architecture and team building.
This is the space to share the things he likes, a couple of ideas and some of his work.