Code Snippets - Sort array of objects by a property in JavaScript
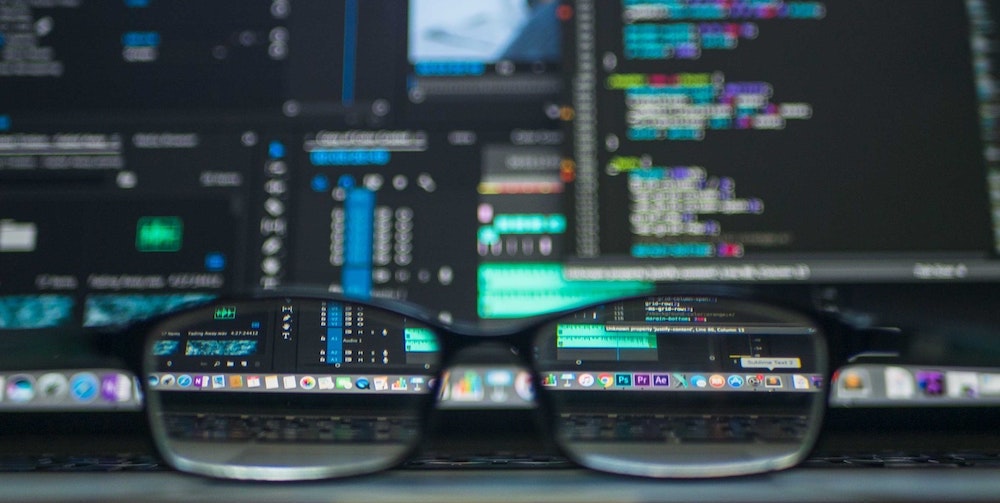
This is another blog post I write about code snippets I find useful. Below is the list of the previous code snippets I have written about:
Today's code snippet is about how to sort and array of objects by a property in JavaScript.
Arrays in JavaScript have a built-in sort method to sort items in ascending order. However, that quite doesn't work out of the box if we'd like to sort an array of integers or objects:
const strArray = ['typescript', 'javascript', 'reactjs', 'nodejs']
strArray.sort()
console.log(strArray) // ["javascript", "nodejs", "reactjs", "typescript"] ā
const numberArray = [1, 10, 2, 100, 3, 1000]
numberArray.sort()
console.log(numberArray) // [1, 10, 100, 1000, 2, 3] ā
const objectArray = [
{
date: new Date(2021, 3, 28),
title: 'Treat Test Code as Production Code',
},
{
date: new Date(2021, 4, 10),
title: 'To-do lists, checkboxes and the definition of done',
},
{
date: new Date(2021, 4, 17),
title: 'Planning the integration of tags in my blog posts',
},
]
objectArray.sort()
console.log(objectArray)
/**
[
{
date: Wed Apr 28 2021 00:00:00,
title: 'Treat Test Code as Production Code'
},ā
{
date: Mon May 10 2021 00:00:00,ā
title: 'To-do lists, checkboxes and the definition of done'
},ā
{
date: Mon May 17 2021 00:00:00,ā
title: 'Planning the integration of tags in my blog posts'
}
]āā
*/
The reason why numberArray
and objectArray
are not property sorted is because the sort
method converts elements to strings and then compares the elements in the array.
This is why all items that start with 1
come first in numberArray
.
The good news is that we can still sort items in the array the way we want.
Especially for objectArray
where we can sort it by either title
or date
properties.
I actually use this to sort blog posts in my blog and you can see the code right here.
The sort
method takes a callback function with 2 arguments we can use to create our custom comparison.
The rule of thumb I use is:
- return
1
if I want to sort items ascending - return
-1
if I want to sort items descending
We can also extract the compare function for reusability:
const numberArray = [1, 10, 2, 100, 3, 1000]
numberArray.sort((itemA, itemB) => (itemA > itemB ? 1 : -1))
console.log(numberArray) // [1, 2, 3, 10, 100, 1000] ā
const objectArray = [
{
date: new Date(2021, 3, 28),
title: 'Treat Test Code as Production Code',
},
{
date: new Date(2021, 4, 10),
title: 'To-do lists, checkboxes and the definition of done',
},
{
date: new Date(2021, 4, 17),
title: 'Planning the integration of tags in my blog posts',
},
]
function sortByName(blogPostA, blogPostB) {
return blogPostA.title > blogPostB.title ? 1 : -1
}
objectArray.sort(sortByName)
/**
[
{
date: Mon May 17 2021 00:00:00,ā
title: 'Planning the integration of tags in my blog posts'
}
{
date: Mon May 10 2021 00:00:00,ā
title: 'To-do lists, checkboxes and the definition of done'
},ā
{
date: Wed Apr 28 2021 00:00:00,
title: 'Treat Test Code as Production Code'
},ā
]āā
*/
I hope that's helpful and interesting to you. šš¼
Did you know you can help me with this page?
If you see something wrong, think this page needs clarification, you found a typo or any other suggestion you might have feel free to open a PR and I will take care of the rest.
My entire site is available to edit on GitHub and all contributions are very welcome š¤š¼.
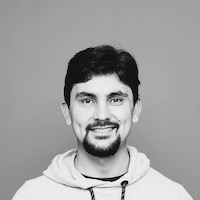
Hemerson Carlin, also known as mersocarlin, is passionate and resourceful full-stack Software Engineer with 10+ years of experience focused on agile development, architecture and team building.
This is the space to share the things he likes, a couple of ideas and some of his work.